arm4::QArmTranReport Class Reference
[ARM 4.0 Transactions]
Transaction instance for application-based and/or remote time measurement. More...
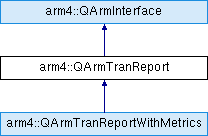
Public Member Functions | |
QArmTranReport (const QArmApplication &app, const QArmTransactionDefinition &definition) | |
Constructs an object that represents an instance of a transaction. | |
const QArmCorrelator & | generateCorrelator () |
Generates a new correlator for the transaction instance. | |
const QArmApplication & | getApplication () const |
returns a reference to the associated application instance. | |
const QString & | getContextURIValue () const |
gets the context URI value. | |
const QString & | getContextValue (int32_t index) const |
gets a context property value. | |
const QArmCorrelator & | getCorrelator () const |
gets the current correlator for this transaction instance. | |
const QArmCorrelator & | getParentCorrelator () const |
gets the correlator of the parent transaction, if any. | |
int64_t | getResponseTime () const |
returns the last response time set on a report() method. | |
int32_t | getStatus () const |
returns the last status value set on a report() method. | |
const QArmTransactionDefinition & | getDefinition () const |
returns a reference the associated transaction definition. | |
const QArmUser & | getUser () const |
returns a reference to the associated user. | |
int32_t | report (int32_t status, int64_t respTimeNanos, int64_t stopTime=QArmConstants::USE_CURRENT_TIME, const QString &diagnosticDetail=NullString) |
provide measurements about a completed transaction. | |
int32_t | setContextURIValue (const QString &value) |
sets the URI context value. | |
int32_t | setContextValue (int32_t index, const QString &value) |
sets a context property value. | |
int32_t | setParentCorrelator (const QArmCorrelator &parent) |
sets the correlator of the parent transaction. | |
int32_t | setUser (const QArmUser &user) |
associates a user, represented by an instance of QArmUser , to the QArmTranReport instance. |
Detailed Description
Transaction instance for application-based and/or remote time measurement.
QArmTranReport
is similar to QArmTransaction
. Both are used to provide data about executing transactions. Instances of both are created based on metadata represented by an QArmTransactionDefinition
, which in turn is scoped by an application definition. Both are scoped by a running application instance, represented by QArmApplication. There are two fundamental differences:
-
With
QArmTransaction
, the response time is measured based onstart()
andstop()
events. WithQArmTranReport
, the application measures the response time, and reports it with a singlereport()
event. -
With
QArmTransaction
, the transaction always executes on the local system in the same process. WithQArmTranReport
, the transaction may execute in the same process, in a different process on the same system, or on a different system.
When executing in the same process, the QArmTranReport
object is created with an QArmApplication
. When executing in a different process on the same system or on a different system, the QArmTranReport
object is created with an QArmApplicationRemote
(a subclass of QArmApplication
).
The two key methods of QArmTranReport are generateCorrelator() and report().
-
generateCorrelator()
generates a new correlator using the immutable data set in the factory method and the current property values set by the four setter methods. It is assumed thatgenerateCorrelator()
is executed zero or once per transaction instance. The practical ramification is that the method implementation will update its internal state to have a unique identifier for an instance (the equivalent ofQArmTransaction
's start handle).getCorrelator()
returns the most recently generatedQArmCorrelator
or QArmCorrelator::Null, ifgenerateCorrelator()
has never been executed. -
report()
is used to provide measurements about a completed transaction. There are two forms. Both provide the status (one of theSTATUS_*
constants inQArmConstants
) and the response time, measured in nanoseconds. One also provides a stop time in the form of milliseconds since January 1, 1970. If a stop time is not provided, or a stop time of -1 (USE_CURRENT_TIME
) is provided, the ARM implementation substitutes the current time; that is, the time when thereport()
method executes. The optional form that takes a string is a way for an application to provide additional diagnostic details when the status is something other thanSTATUS_GOOD
.
As noted above, generateCorrelator()
updates the internal state for a new transaction instance. The first time report()
executes after generateCorrelator()
, report()
will not update the internal state for a new transaction instance; it will use the instance identifier from the generateCorrelator()
. If report()
executes twice in succession, or if generateCorrelator()
has never been executed, report()
will update the internal state for a new transaction instance. Summarizing, there are two patterns:
-
If correlators for the current transaction are not requested,
generateCorrelator()
is not used.report()
is executed after each instance completes, and each time it generates a new instance identifier, like a start handle. -
If correlators for the current transaction are requested,
generateCorrelator()
andreport()
are used in pairs. FirstgenerateCorrelator()
establishes the transaction instance identifiers, as well as creating a correlator. This correlator is sent to downstream transactions. After the downstream transactions complete, and the current instance completes,report()
provides the measurements. In this casereport()
does not update the transaction instance identifier.
In addition to the identity properties from QArmApplication
and QArmTransactionDefinition
, there are four optional setter methods to establish additional instance-level context. They can be used at any time to update the attribute within the object. The only time the properties are meaningful is when generateCorrelator()
or report()
executes. At the moment either method executes, the current values are used, any or all of which may be null. See the description provided for the individual methods below.
- Note:
QArmTranReport
should only be used within a single thread. It does not make much sense to use an instance of this class across threads thus no locking is implemented.
Constructor & Destructor Documentation
arm4::QArmTranReport::QArmTranReport | ( | const QArmApplication & | app, | |
const QArmTransactionDefinition & | definition | |||
) |
Constructs an object that represents an instance of a transaction.
The metadata is supplied in an QArmTransactionDefinition
object. It is scoped by an application instance, represented by QArmApplication
(or its subclass, QArmApplicationRemote
).
- Parameters:
-
app the application instance the transaction belongs to. definition the metadata describing the type of the transaction.
Member Function Documentation
const QArmCorrelator& arm4::QArmTranReport::generateCorrelator | ( | ) |
Generates a new correlator for the transaction instance.
See the discussion of this method in the interface description above.
- Returns:
- a new
QArmCorrelator
const QArmApplication& arm4::QArmTranReport::getApplication | ( | ) | const |
returns a reference to the associated application instance.
- Returns:
- the
QArmApplication
used in the constructor to create this instance ofQArmTranReport
.
const QString& arm4::QArmTranReport::getContextURIValue | ( | ) | const |
gets the context URI value.
- Returns:
- the context URI value, or
null
const QString& arm4::QArmTranReport::getContextValue | ( | int32_t | index | ) | const |
gets a context property value.
See the description of setContextValue().
- Parameters:
-
index index into the array aof context properties.
- Returns:
- the value at
index
, ornull
.
const QArmCorrelator& arm4::QArmTranReport::getCorrelator | ( | ) | const |
gets the current correlator for this transaction instance.
See the discussion in the interface description above for when the state of the instance affecting the correlator is updated.
- Returns:
- an
QArmCorrelator
for this transcation instance.
const QArmTransactionDefinition& arm4::QArmTranReport::getDefinition | ( | ) | const |
returns a reference the associated transaction definition.
- Returns:
- the
QArmTransactionDefinition
used in the constructor.
const QArmCorrelator& arm4::QArmTranReport::getParentCorrelator | ( | ) | const |
gets the correlator of the parent transaction, if any.
- Returns:
- the last value set with setParentCorrelator(). The vaue may be a
QArmCorrelator::Null
.
int64_t arm4::QArmTranReport::getResponseTime | ( | ) | const |
int32_t arm4::QArmTranReport::getStatus | ( | ) | const |
returns the last status value set on a report()
method.
If report()
has never executed, it returns STATUS_INVALID
.
- Returns:
- one of the status values defined in QArmConstants.
const QArmUser& arm4::QArmTranReport::getUser | ( | ) | const |
returns a reference to the associated user.
- Returns:
- the user associated with this transaction instance, if set with setUser(), or
QArmUser::Null
.
int32_t arm4::QArmTranReport::report | ( | int32_t | status, | |
int64_t | respTimeNanos, | |||
int64_t | stopTime = QArmConstants::USE_CURRENT_TIME , |
|||
const QString & | diagnosticDetail = NullString | |||
) |
provide measurements about a completed transaction.
See the discussion of this method in the interface description above.
- Parameters:
-
status transaction status. See QArmConstants. respTimeNanos response time (in nanoseconds) stopTime stop time (milliseconds since January 1, 1970) diagnosticDetail additional details, used when status other than QArmConstants::STATUS_GOOD
- Returns:
- 0 on sucess; otherwise, a non-zero error code is returned (as specified in QArmInterface).
int32_t arm4::QArmTranReport::setContextURIValue | ( | const QString & | value | ) |
sets the URI context value.
- Parameters:
-
value the new URI context value.
- Returns:
- 0 on sucess; otherwise, a non-zero error code is returned (as specified in QArmInterface).
int32_t arm4::QArmTranReport::setContextValue | ( | int32_t | index, | |
const QString & | value | |||
) |
sets a context property value.
This method sets one of the maximum 20 context properties that may change for each transaction instance. The context property name at the specified array index must have been set to a non-NullString
value when the QArmTransactionDefinition
object was created. If the name is a NullString
, the value will be set to NullString
.
- Parameters:
-
index index into the array of context properties. value the new context value.
- Returns:
- 0 on sucess; otherwise, a non-zero error code is returned (as specified in QArmInterface).
int32_t arm4::QArmTranReport::setParentCorrelator | ( | const QArmCorrelator & | parent | ) |
sets the correlator of the parent transaction.
- Parameters:
-
parent an QArmCorrelator object referring to the parent transaction, or QArmCorrelator::Null
.
- Returns:
- 0 on sucess; otherwise, a non-zero error code is returned (as specified in QArmInterface).
int32_t arm4::QArmTranReport::setUser | ( | const QArmUser & | user | ) |
associates a user, represented by an instance of QArmUser
, to the QArmTranReport instance.
This user is assumed to be the user for all start()
/ stop()
pairs until the association is changed or cleared. setUser(QArmUser::Null)
clears any existing association to an QArmUser
.
- Parameters:
-
user an QArmUser
, orQArmUser::Null
- Returns:
- 0 on sucess; otherwise, a non-zero error code is returned (as specified in QArmInterface).
The documentation for this class was generated from the following file:
- QArm4/QArmTranReport